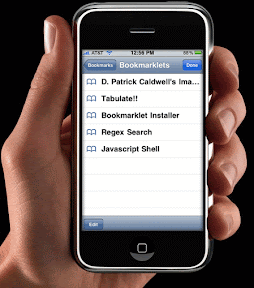
This bookmarklet is probably my most used bookmarklet. Basically, you enter a regular expression and each match in the page will be highlighted. It cycles through 16 color schemes to change the highlight color.
If you just want to install the bookmarklet, grab this link and drag it onto your bookmarklet toolbar in your browser.
Regex Search
If you want to use it on your mobile device, you can use my Mobile Bookmarklet Installer Bookmarklet (which, by the way, will install itself too).
I'm sure it doesn't work in IE, but I haven't tested it in a really long time. If you'd like to see what it would do in IE if IE didn't suck so badly, just click it.
If you're interested in the code, here it is!
// check to see if the variable searches has been defined. // if not, create it. this variable is to cycle through // highlight colors. if (typeof(searches) == 'undefined') { var searches = 0; }; ( function() { // just some variables var count = 0, text, regexp; // prompt for the regex to search for text = prompt('Search regexp:', ''); // if no text entered, exit bookmarklet if (text == null || text.length == 0) return; // try to create the regex object. if it fails // just exit the bookmarklet and explain why. try { regexp = new RegExp(text, 'i'); } catch (er) { alert('Unable to create regular expression using text \'' + text + '\'.\n\n' + er); return; } // this is the function that does the searching. function searchWithinNode(node, re) { // more variables var pos, skip, acronym, middlebit, endbit, middleclone; skip = 0; // be sure the target node is a text node if (node.nodeType == 3) { // find the position of the first match pos = node.data.search(re); // if there's a match . . . if (pos >= 0) { // create the acronym node. acronym = document.createElement('ACRONYM'); acronym.title = 'Search ' + (searches + 1) + ': ' + re.toString(); acronym.style.backgroundColor = backColor; acronym.style.borderTop = '1px solid ' + borderColor; acronym.style.borderBottom = '1px solid ' + borderColor; acronym.style.fontWeight = 'bold'; acronym.style.color = borderColor; // get the last half of the node and cut the match // out. then, clone the middle part and replace it with // the acronym middlebit = node.splitText(pos); endbit = middlebit.splitText(RegExp.lastMatch.length); middleclone = middlebit.cloneNode(true); acronym.appendChild(middleclone); middlebit.parentNode.replaceChild(acronym, middlebit); count++; skip = 1; } } // if the node is not a text node and is not // a script or a style tag then search the children else if ( node.nodeType == 1 && node.childNodes && node.tagName.toUpperCase() != 'SCRIPT' && node.tagName.toUpperCase != 'STYLE' ) for (var child = 0; child < node.childNodes.length; ++child) child = child + searchWithinNode(node.childNodes[child], re); return skip; } // use the search count to get the colors. var borderColor = '#' + (searches + 8).toString(2).substr(-3) .replace(/0/g, '3') .replace(/1/g, '6'); var backColor = borderColor .replace(/3/g, 'c') .replace(/6/g, 'f'); // for the last half of every 16 searhes, invert the // colors. this just adds more variation between // searches. if (searches % 16 / 8 >= 1) { var tempColor = borderColor; borderColor = backColor; backColor = tempColor; } searchWithinNode(document.body, regexp); window.status = 'Found ' + count + ' match' + (count == 1 ? '' : 'es') + ' for ' + regexp + '.'; // if we made any matches, increment the search count if (count > 0) searches++; } )();
Well, This is an interesting software which is like by many people and some of the people would like to learn more from here. I really enjoy the information you have posted here. Thank you so much for posting this article. free download software for pc
ReplyDeleteThis article has many new features which shares many valuable things. Thank you so much for posting this article. full version
ReplyDeleteWell, what is the main purpose of this software. please explain here. free download software for pc
ReplyDeleteJavaScript Shell Code is amazing ...... Thanks for this code...
ReplyDeleteFREE DOWNLOAD SOFTWARE | FULL VERSION
The great post about JavaScript, thanks a lot for sharing.
ReplyDeletefull version software blogspot sites
Good sharing! thanks for knowledgeable posting for bookmarklets.
ReplyDeletePC Software Download
valuable post! very interesting and helpful sharing!
ReplyDeleteSoftware Crack | Crack Software Download
thanks for useful sharing.
ReplyDeleteFull Crack Software
I really enjoy the information you have shared. Thank you so much..!!
ReplyDeleteSoftware Crack | Free Download Software
This is one of the amazing information. thanks a lot for sharing...!!!!
ReplyDeleteSoftware Full Version | Download Free Software
How to use it.?
ReplyDeletecrack software download | fonepaw registration key
That was it i am searching for.
ReplyDeletewavepad registration code | magic bullet serial
I am glad that you shared this helpful info with us.
ReplyDeleteidealshare videogo serial key | kastor all video downloader full crack
thanks a lot.its valuable sharing!
ReplyDeletedxo viewpoint 2 activation code
Subscribe me -> http://ggmalgues.890m.com/
ReplyDeleteHad to remove all the comments from the script and prefix it with "javascript:" but now it's working excellently. Powerful and impressive little bookmarklet.
ReplyDelete